How to create CheckBox onClick Event in asp.net MVC
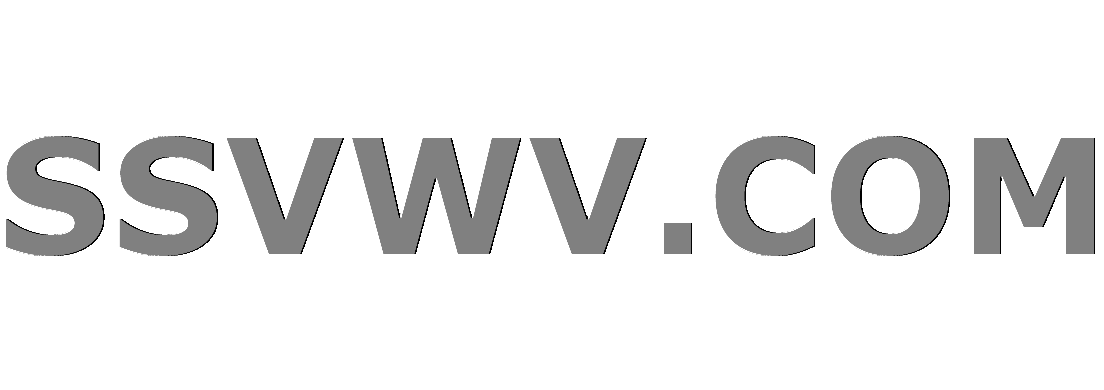
Multi tool use
How to create CheckBox onClick Event in asp.net MVC
I'm accessing the data for the CheckBoxes from the database, now I want to display the form for each and every checkbox if it's checked. How to achieve this?
My form design -
<div class="col-sm-12" id="roomtypes">
@foreach(var item in ViewBag.RoomTypes)
{
<input type="checkbox" id="chk_@item.id" name="RoomTypes" value="@item.id" /> @item.type<br />
<div class="col-sm-12" style="display:none;" id="Price_@item.id">
<input type="number" placeholder="Enter Price" id="PriceValue" />
</div>
}
</div>
I want to display the price input when checkbox is checked.
My back-end code -
ViewBag.RoomTypes = db.RoomTypes.ToList();
My code's generating this output -
My code's generating this output
Can u guide me how to do that for each check-boxes generated. Please.
– Gautam Sharma
Jun 30 at 2:23
2 Answers
2
You can easily do that with css
. Add chkshowhide
css class to your input and div and add css
as per code. Your code will be as below.
css
chkshowhide
css
<div class="col-sm-12" id="roomtypes">
@foreach(var item in ViewBag.RoomTypes)
{
<input type="checkbox" id="chk_@item.id" name="RoomTypes" value="@item.id" class="chkshowhide"/> @item.type<br />
<div class="col-sm-12 chkshowhide" id="Price_@item.id">
<input type="number" placeholder="Enter Price" id="PriceValue" />
</div>
}
</div>
css.
div.chkshowhide {
display: none;
}
input.chkshowhide:checked + br + div.chkshowhide {
display: block;
}
You can test sample here.
function saveData() {
$('input.chkshowhide:checked').each(function() {
var chkValue = $(this).val();
var divId = this.id.replace("chk", "Price");
var priceValue = $('#' + divId).find('input').val();
console.log('chkValue=' + chkValue + ", priceValue=" + priceValue);
// Write your save code here
});
}
div.chkshowhide {
display: none;
}
input.chkshowhide:checked + br + div.chkshowhide {
display: block;
}
<script src="https://ajax.googleapis.com/ajax/libs/jquery/1.7.1/jquery.min.js"></script>
<input type="checkbox" id="chk_1" name="RoomTypes" value="1" class="chkshowhide"> 1<br />
<div class="col-sm-12 chkshowhide" id="Price_1">
<input type="number" placeholder="Enter Price" id="PriceValue" />
</div>
<input type="checkbox" id="chk_2" name="RoomTypes" value="2" class="chkshowhide"> 2<br />
<div class="col-sm-12 chkshowhide" id="Price_2">
<input type="number" placeholder="Enter Price" id="PriceValue" />
</div>
<input type="checkbox" id="chk_3" name="RoomTypes" value="3" class="chkshowhide"> 3<br />
<div class="col-sm-12 chkshowhide" id="Price_3">
<input type="number" placeholder="Enter Price" id="PriceValue" />
</div>
<input type="button" value="Save" onclick="saveData();" />
Thanks @Karan it worked! I appreciate your help.
– Gautam Sharma
Jun 30 at 13:27
Now suppose, I need to save these details individually (one by one - depends on the number of check-boxes checked) to database, how to do that in MVC?
– Gautam Sharma
Jun 30 at 14:42
@GautamSharma Updated code for Save one by one data for each checked input.
– Karan
Jun 30 at 16:09
Thank you for your help @Karan
– Gautam Sharma
Jun 30 at 17:01
Here's a jQuery example.
First, add a helper class to the html. Let's also encode the identifier we can pick-up in the JavaScript handler data-id
.
data-id
<input class="roomtype_chk" data-id="@item.id"
type="checkbox" id="chk_@item.id" name="RoomTypes" value="@item.id" />
Instead of an inline display style let's make that a class also "hidden".
<div class="col-sm-12" id="Price_@item.id" class="hidden">
<input type="number" placeholder="Enter Price" id="PriceValue" />
</div>
If you're using Bootstrap that comes installed with the template then the class style already defined for you. If not then
.hidden { display: none; }
Now, you can use a selector to quickly bind all specified checkboxes
$(".roomtype_chk").on("click", function(e)
{
var myValue = $(this).value();
var id = $(this).attr("data-id");
$("#Price_" + id).removeClass("hidden");
});
Beware:
id
@foreach
@for
name
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Bind a JavaScript event to the checkbox input. When the checkbox value changes, test the checkbox value, then make your price input visible or attach a new input (created in JavaScript) to your form.
– Jasen
Jun 29 at 17:48