Access all holder views inside OnBindViewHolder in RecyclerView
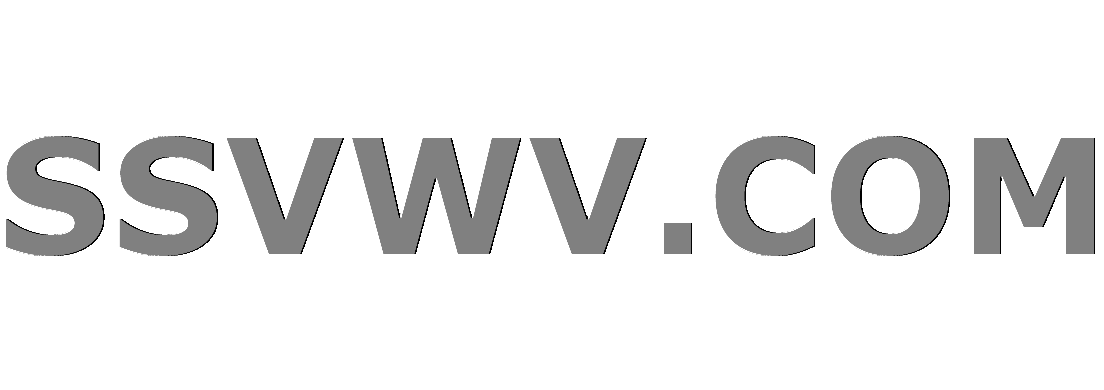
Multi tool use
Access all holder views inside OnBindViewHolder in RecyclerView
I need to set the Clickable property of all the cards in a recycler view (with RecyclerView adapter class) to false once I click on any one of the cards. How do I do that? Setting holder.cardView.setClickable(false) only does it for the clicked one. How do I access all the cards in the view in OnBindViewHolder?
4 Answers
4
You cannot do that due to the way RecyclerView
lazy load the child view. The child views are only created and rendered when they are visible on the screen, other views are cleared to save memory.
RecyclerView
You can do these to have the same effect:
1/ Create an overlay FrameLayout
on top of your RecyclerView
, set its clickable
to true, then show/hide it when needed to prevent touch
FrameLayout
RecyclerView
clickable
<FrameLayout>
<RecyclerView ... />
<FrameLayout
android:id="@+id/overlay_view"
...
android:clickable="true" />
</FrameLayout>
cardItem.setOnClickListener(view -> {
// The mOverlayView will intercept all the touch event on RecyclerView
mOverlayView.setVisibility(View.VISIBLE);
})
2/ Create a boolean preventClick
variable, set it to true when you want to prevent user's click. Then check for this value in your item's OnClickListener.
boolean preventClick
You want to prevent click on current visible items, but what if user scroll down a bit, visible items will changed, then click one of them. I may understand you wrong but the above approach are what I will do. An overlay view will intercept all the click if you make it visible when any card is clicked
– Tam Huynh
Jun 30 at 9:54
My idea is:
In your adapter class define:
public static SparseBooleanArray itemCardClickState = new SparseBooleanArray();
private int currentPlayingPosition = -1;
and in your viewHolder class:
myCardItem..setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int position = getAdapterPosition();
int prev = currentPlayingPosition;
currentPlayingPosition = position;
if (prev >= 0)
notifyItemChanged(prev);
// refresh previously clicked item view
itemCardClickState .put(currentPlayingPosition, true);
}
});
and check the card state in onBinViewHoldee is true or false
I found a long process which solves your problem with a clean code
Step 1 - Take your item click listener to the Activity with an interface. Add this code to your RecyclerView Adapter
private RecyclerViewItemClickListener recyclerViewItemClickListener;
public void setRecyclerViewItemClickListener(RecyclerViewItemClickListener recyclerViewItemClickListener) {
this.recyclerViewItemClickListener = recyclerViewItemClickListener;
}
public interface RecyclerViewItemClickListener {
void onClick(View v);
}
Step 2 - Make the click listener for your item in the ViewHolder, not in the onBindViewHolder
public class ViewHolder extends RecyclerView.ViewHolder {
public ViewHolder(View view) {
super(view);
view.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
recyclerViewItemClickListener.onClick(v);
}
});
}
}
In your Activity, use the interface to set click listener and on click override it again with a blank code.
adapter.setRecyclerViewItemClickListener(new RecyclerViewAdapter.RecyclerViewItemClickListener() {
@Override
public void onClick(View v) {
//do everything that should happen on click
//then set a blank click listener
adapter.setRecyclerViewItemClickListener(new RecyclerViewAdapter.RecyclerViewItemClickListener() {
@Override
public void onClick(View v) {
}
});
}
});
I tested it and it works as you want it to
Declare a Integer
variable in adapter and assign the corresponding postion to which positioned view is enabled and compare the value in bindItem
and enable, disable according. Also make sure to call notifyDataSetChanged()
when clicked.
Integer
bindItem
notifyDataSetChanged()
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Yes, I want only the existing child cards that are currently in view to be unclickable, for e.g., I have 4 cards visible at a time, so all of them should be unclickable, if I click any one of them to avoid double clicking
– Bitten Tech
Jun 30 at 9:47