Accessing a private field from one class to another not related to the former class in C++ (OOP)
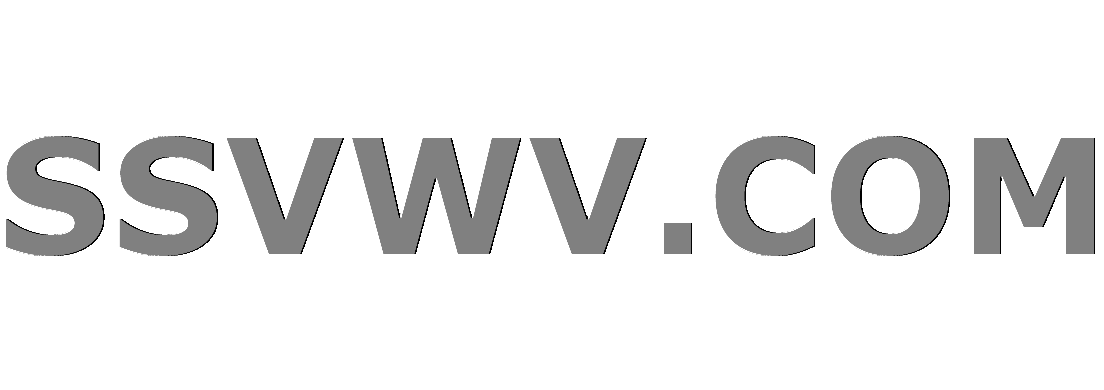
Multi tool use
Accessing a private field from one class to another not related to the former class in C++ (OOP)
I have 2 classes - A and B
. Class A
has a member list<int>
and a method addData()
that adds a number to this list. The second class B
has a function displayContent()
- it iterates through the list of the class A
and prints its content (the added numbers). The problem I face is that I cannot access the private
(cannot be changed to public) field list
of class A
from the newly instantiated object (from class B
). I would like to ask how can this be achieved with getters/setters
, or a copy constructor
?
A and B
A
list<int>
addData()
B
displayContent()
A
private
list
A
B
getters/setters
copy constructor
//identifier 'a' is undefined?
a
4 Answers
4
In displayContent_a, your identified 'a' is undefined, because within that function or class you have never declared a variable 'a'. A simple solution is to pass an object of type A to the function which determines what A object to display the list of:
void displayContent_a(A& a) {
//this uses the a from the parameter
for (auto x = a.a.begin(); x != a.a.end(); x++) { //use a.a because we want to access the list
cout << *x << endl;
}
}
However, this won't be enough, as B can't access private data of A. To fix this, you have to make B a friend of A. This can be done in the following way:
class B; //forward declare B so it can be used in friend declarations in A
class A
{
//... your content
friend class B;
}
Removed my answer. He asks it to be done with a copy constructor, which is impossible as far a I know.
– user4035
Jun 30 at 7:35
I don't see how a copy constructor would be involved with this problem.
– MivVG
Jun 30 at 7:39
In good code classes are never entangled. This means that if class B
uses class A
than class A
shouldn't have any knowledge about B
.
So adding a friendship is just a workaround which makes code entangled.
B
A
A
B
It is hard to say what is best approach without knowing more context, but there are couple possibilities.
You can add such API to A:
class A {
public:
void forEach(std::function<void(int)> f) const {
for(auto x : mData) {
f(x);
}
}
private:
list<int> mData;
};
class B {
public:
void displayContent(std::ostream &out) {
a.forEach([&out](int x){ out << x<< ", "; });
}
private:
A a;
};
Advantage is that you can change lots of thing in A
(for example change list to vector) and you do not have to fix anything in B
.
A
B
There are more possibilities. Simple getter could solve issue:
class A {
public:
list<int> getData() const {
return mData;
}
private:
list<int> mData;
};
To access variable 'a' of class A, it is not possible with current implementation.
Firstly, make class B as the derived class of base class A
Even then, it is not possible to access the private variable of class A from anywhere outside even from derived class.
Try to implement by declaring variable as protected.
Another implementation can be by declaring class B as friend of class A.
That way, private variables can be accessed from class B
A getter is a member function which allows the member attribute List<int> L
to be read from outside of the object.
In class A define:
List<int> L
public:
List<int> getList(){
return L;
}
Now you can add In Class B:
A a(constructor args);
List<int>=a.getList();
in the same measure you can use setters to change the value of private attributes e.g
public:
void setList(List<int> data){
L=data;
}
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
//identifier 'a' is undefined?
- you didn't pass an instance of A as a variable and B is not a child of A, so how cana
be defined?– user4035
Jun 30 at 7:39