Angular Yahoo Weather API
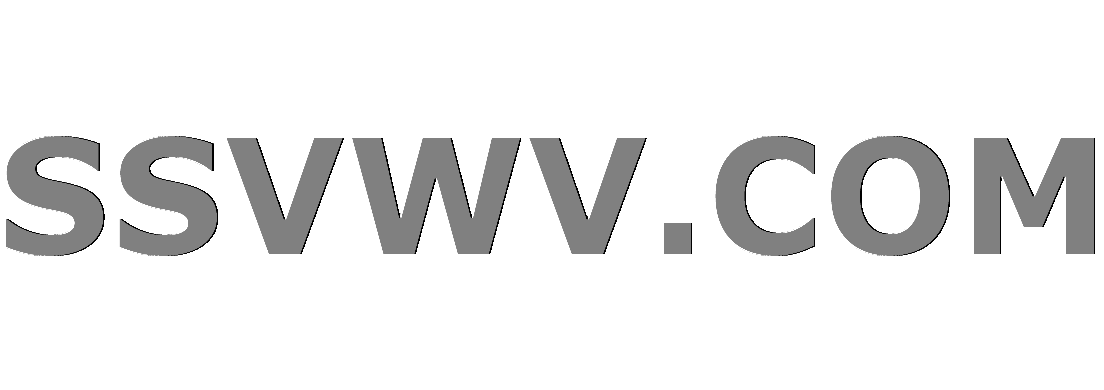
Multi tool use
Angular Yahoo Weather API
I have a problem with the Yahoo Weather API. How should I make a request to get data from the Yahoo Weather API?
import { Injectable } from '@angular/core';
import {Observable} from 'rxjs';
import {HttpClient} from '@angular/common/http';
@Injectable()
export class WeatherService {
constructor(private http: HttpClient) { }
getWeatherForecast(city: string): Observable<any> {
const url = 'https://query.yahooapis.com/v1/public/yql?q=select wind from
weather.forecast where woeid=2460286';
return this.http.get(url);
}
}
You're currently getting a 404, possibly because of spaces in the URL. Can you try using
encodeURI
on that url, and using the encoded URL instaed? Also, when I hit that URL, I'm getting XML back, which may cause you issues, as HttpClient will try to parse the results as JSON by default– user184994
Jun 30 at 8:41
encodeURI
I have changed url for query.yahooapis.com/v1/public/yql?q=select%20*%20from%20weather.forecast%20where%20woeid%20in%20(select%20woeid%20from%20geo.places(1)%20where%20text%3D%22greenland%22)&format=json&env=store%3A%2F%2Fdatatables.org%2Falltableswithkeys from docs, but result is same.
– gladki24
Jun 30 at 8:44
If you check in your browser's developer tools network panel, is it trying to connect to the correct URL? That URL seems to work fine for me? Failing that, can you please create a StackBlitz that reproduces the issue? Thanks
– user184994
Jun 30 at 8:47
You are right. I have In-memory-web-api in app. I think it is a problem.
– gladki24
Jun 30 at 8:56
2 Answers
2
Working example: stackblitz
Your only problem was that you forgot to add: &format=json to your url string.
The returned data was in XML format by default.
This is a simple example, but I hope it helps you. I'll use a library called axios
right here:
axios
const url = "https://query.yahooapis.com/v1/public/yql?q=select item.condition from weather.forecast where woeid in (select woeid from geo.places(1) where text='Sunderland') and u='c'&format=json";
const getWeatherData = () => {
axios.get(url)
.then(res => console.log(res.data.query.results.channel.item.condition))
.catch(err => console.log(err.data))
}
getWeatherData();
<script src="https://cdnjs.cloudflare.com/ajax/libs/axios/0.12.0/axios.min.js"></script>
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Please edit your question with the actual code instead of a picture. Thank you
– user184994
Jun 30 at 8:30