How does innerHTML actually work when set to resultant (result of array.filter) array?
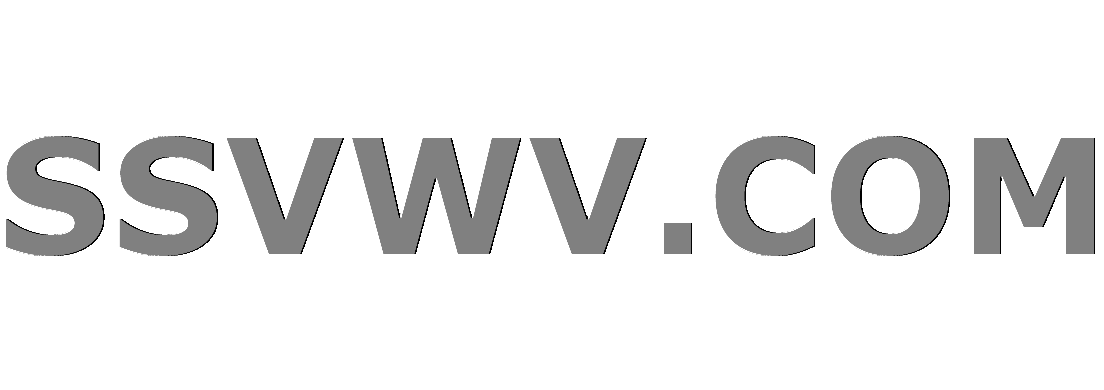
Multi tool use
How does innerHTML actually work when set to resultant (result of array.filter) array?
In the following fiddle, JS Fiddle, filter returns all those element that pass the test (age >= 18)
. I have couple of doubts in this regards:
(age >= 18)
Why does array.filter
return array elements even though I've explicitly defined a return value the callback return, here, an object's property user.name
array.filter
user.name
How does innerHTML
actually works in this case, since the result_arr
is actually an array object (typeof result_arr
) and AFAIK, values needs to be first extracted from result_arr
and then written as the target elements content. It looks like, JavaScript implicitly
does this for us?
innerHTML
result_arr
typeof result_arr
result_arr
JavaScript implicitly
JS
function myFunction() {
var result_arr = ages.filter(checkAdult);
document.getElementById('demo').innerHTML = result_arr
}
var user = {
name: 'Nick',
age: 50
}
var ages = [32, 33, 16, 40];
function checkAdult(age) {
if(age >= 18){
return user.name
}
}
function myFunction() {
var result_arr = ages.filter(checkAdult);
document.getElementById('demo').innerHTML = result_arr
}
HTML
<button onclick="myFunction()">Try it</button>
<p id="demo"></p>
Array.filter
innerHTML
3 Answers
3
Why does array.filter
return array elements even though I've explicitly defined a return value the callback return, here, an object's property user.name
array.filter
user.name
Because that's how filter
works. All it cares about is whether the return value of the callback is falsy¹ or truthy. If you want to use a different value in the result array, you want map
. It's common to see .filter(...).map(...)
(common enough I've been known to create a .filterMap
utility function).
filter
map
.filter(...).map(...)
.filterMap
How does innerHTML
actually works in this case, since the result_arr
is actually an array object
innerHTML
result_arr
result_arr
is coerced to string, as with String(result_arr)
. With arrays, coercing to string is the same as .join()
, which is the same as .join(",")
.
result_arr
String(result_arr)
.join()
.join(",")
¹ "falsy" and "truthy": A value that coerces to false
when used in a condition (e.g., as a boolean) is a falsy value. The falsy values are 0
, ""
, NaN
, null
, undefined
, and of course, false
. All other values are truthy.
false
0
""
NaN
null
undefined
false
Why does array.filter return array elements even though I've explicitly defined a return value the callback return, here, an object's property user.name
Because filter expects a boolean return type. If it's true, the element stays, if it's false, it doesn't.
See mdn: The filter() method creates a new array with all elements that pass the test implemented by the provided function.
How does innerHTML actually works in this case, since the result_arr is actually an array object (typeof result_arr) and AFAIK, values needs to be first extracted from result_arr and then written as the target elements content. It looks like, JavaScript implicitly does this for us?
Array.prototype.toString()
will be implicitly called for you, creating a comma separated string. This behavior is defined here in the spec
Array.prototype.toString()
Right @Teemu, I've been downvoted quite some times for stating toString() is called, but I've been investigating it further the same time and the spec says it's toString() - more exactly its
toPrimitive
that get's "called", that results in a call to toString for an array– bambam
Jun 30 at 9:56
toPrimitive
Here's more ecma-international.org/ecma-262/5.1/#sec-9.1 @Teemu
– bambam
Jun 30 at 9:58
toString
is definitely what is used here, and stringifying an object in general. Voting at SO is nowadays weird. Blatantly wrong answers get upvotes, also totally dumb and/or off-topic questions get upvotes, and when you say something which is not obvious (but correct), you'll get down votes, unless you don't add a list of links to the specs too = ).– Teemu
Jun 30 at 10:04
toString
Yeah, it's a bit strange. Especially for someone who's self learning and a bit dependent on others input, the voting behavior here is confusing... @Teemu I don't care too much about the points, but it's a good indicator to how I did understand what I've been learning...+
– bambam
Jun 30 at 10:05
In addition to the other answers, if you want to filter and transform an array in one go (without iterating over the array twice), you can use reduce
:
reduce
var ages = [32, 33, 16, 40];
var result_arr = ages.reduce((a, item) => {
if (item >= 18) a.push(item + 0.5);
return a;
}, );
console.log(result_arr);
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Array.filter
returns an array, always.innerHTML
stringifies whatever you pass to it, always, and with arrays it just joins it together in the default way– adeneo
Jun 30 at 9:24