How to dispatch fetched data from API?
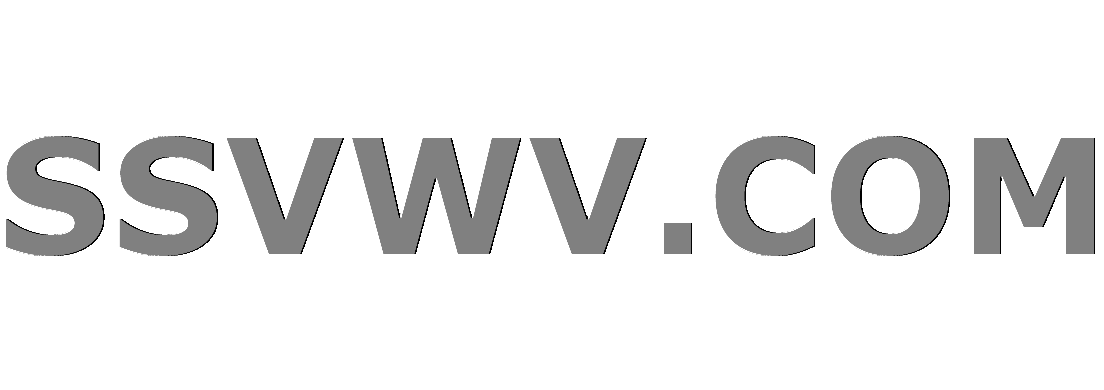
Multi tool use
How to dispatch fetched data from API?
I have fetchWeatherData(location)
- this file fetch data from weather API
fetchWeatherData(location)
API
When I call store.dispatch(fetchWeatherData('london'))
all works correctly
store.dispatch(fetchWeatherData('london'))
But, how can I do this without store.dispatch
...? I mean automaticly
store.dispatch
1 Answer
1
I think you are looking for something called mapDispatchToProps
.
mapDispatchToProps
const mapDispatchToProps = (dispatch) => ({
fetchWeatherData: (payload) => {
dispatch(fetchWeatherData(payload))
}
});
Now you need to connect
this to your component and use it inside this.
connect
class Test extends React.Component {
componentWillMount () {
this.props.fetchWeatherData("london")
}
render () {
return null;
}
}
const withStore = connect(null, mapDispatchToProps);
export default withStore(Test);
You can read more about this in redux documentation.
By clicking "Post Your Answer", you acknowledge that you have read our updated terms of service, privacy policy and cookie policy, and that your continued use of the website is subject to these policies.
Check: redux.js.org/api-reference/bindactioncreators
– Shota
Jun 30 at 8:59